Date tip published: | 12/05/2005 |
Description: | A JavaBean is a reusable component that can be used in any Java application development environment. JavaBeans are dropped into an application container, such as a JavaServer Page, and can perform functions ranging from a simple animation to complex calculations. A JavaBean is just a Java class that conforms to the JavaBean coding guidelines. Unlike tag libraries or servlets, there is no requirement to extend or implement a "bean" class to produce a JavaBean. If your Java code is developed as a bean then it is easier to use (and re-use) that bean in other Java applications like JavaServer Pages, servlets, and Java programs. |
To learn more about Java and WebSphere development use the following links:
Introduction to WebSphere Using Rational Developer 6
Introduction to WebSphere for Domino Developers using Rational Developer 6
Servlet and JSP Programming for WebSphere using Rational Developer 6
Servlet and JSP Programming using Rational Developer 6 for Domino Developers
Creating JavaBeans in Rational Developer 6
There are many aspects of Java programming that can take advantage of JavaBeans.
JavaBeans or just "Beans" are used in many types of Java applications, such as:
- Java GUI client applications
- Web Services
- Servlets
- JavaServer Pages
- Enterprise JavaBeans
It is considered a best practice to code new Java object classes as JavaBeans whenever possible.
The JavaBean API
The definition of a JavaBean from Sun Microsystems is: "A reusable software component that can be manipulated visually in a builder tool". This is a rather broad definition. JavaBeans were introduced to be plug-ins to software development tools referred to as "Bean Builders" or "Bean Boxes". These software tools allow non-Java programmers to implement "beans" (reusable components) without having to understand the complexity of the underlying Java code. Using these builders, developers could easily "wire" together beans such as a user interface bean, a network bean and a data bean to rapidly develop a complete application. A JavaBean's methods are exposed to builder tools like Rational Developer and Borland's JBuilder.
But what is a JavaBean? A JavaBean is a Java class that conforms to the JavaBean coding guidelines. Unlike tag libraries or servlets, there is no requirement to extend or implement a "bean" class to produce a JavaBean. The JavaBean's component model was introduced in the Java 1.1 SDK. Java 1.2 extended that model by introducing a containment and services protocol. Although there is a formal JavaBean API, the API is really not the most important part of implementing and using JavaBeans. The JavaBean's component model relies on a number of rules and conventions which bean developers must follow. The conventions are sometimes referred to as design patterns. For example there are conventions or patterns for naming methods to access the properties defined by a bean.
JavaBean Coding Guidelines
A JavaBean is just a Java class that conforms to the JavaBean coding guidelines. The JavaBean class file should conform to the following guidelines:

Component | 
Guideline |

Class Name | 
There are no restrictions on the class name of a bean. |

Superclass | 
A bean can extend any other Java class. Beans are often AWT or Swing components, but there are no restrictions. |

Instantiation | 
A bean must provide a no-parameter constructor. If the bean class does not define a constructor, then the no-parameter constructor is automatically used by default. |

Bean Name | 
The name of a bean is the name of the class that implements the bean. |

Method Names | 
Methods must be declared public. A method can have any name that does not conflict with the property and event-naming conventions of getMethodName and setMethodName. The name should be as descriptive as possible. |

Field Variable Names | 
Field variables are declared private and can only be accessed through the appropriate getter and setter methods. Field variable names should always start with a lower case character. |
The following template can be used for a basic JavaBean. The getter and setter methods return the data type of the corresponding field variable.
public class BasicBean { |
private dataType fieldVariable; |
public BasicBean() { |
// no-parameter constructor |
} |
public void setFieldVariable(){ |
this.fieldVariable = fieldVariable; |
} |
public dataType getFieldVariable(){ |
return fieldVariable; |
} |
} |
Field and Method Names in JavaBeans
A field variable name should always start with a lower case character. In the getter and setter methods, the field name is proper cased with the "get" or "set" added to the beginning of the field name.
private int age; |
public int getAge() { |
return age; |
} |
public void setAge(int i) { |
age = i; |
} |
Boolean values are handled differently then other native data types. Instead of using the "get" prefix to return the value of a boolean variable, the "is" prefix is used to form a question. The "set" prefix is still used to set the value.
private boolean status; |
public boolean isStatus() { |
return status; |
} |
public void setStatus(boolean boolean1) { |
status = boolean1; |
} |
|
|
Creating a JavaBean using the Wizard in Rational Developer
Rational Developer provides a wizard to create a JavaBean and to generate the getter and setter methods for the bean automatically. This can save time and reduce errors. The programmer creates the JavaBean but only types in the field variables that will be used. A wizard can then be used to generate the getter and setter methods. The programmer can then add code to complete the methods.
Procedure: Create a JavaBean using the Wizard
Follow these steps to create a JavaBean using the wizard:
- Open the Package Explorer view in the Java Perspective.
- Right click the package for the JavaBean class and select New | Class from the context menu. Provide a name for the class and make sure that 'public static void main(String[] args)' option is not checked. Click the [Finish] button to create the JavaBean class.
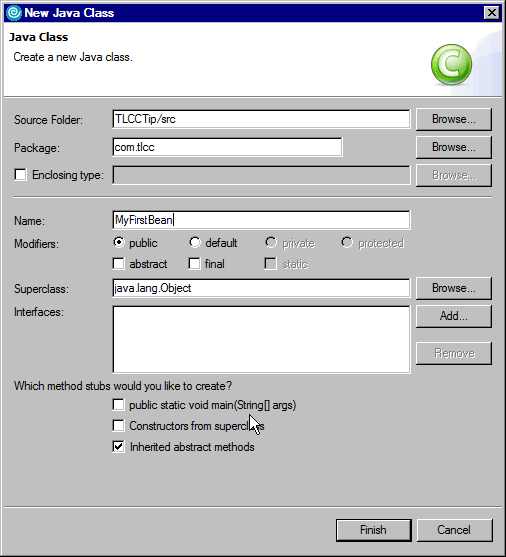
- In the body class, add private field variables for each piece of data to be manipulated.
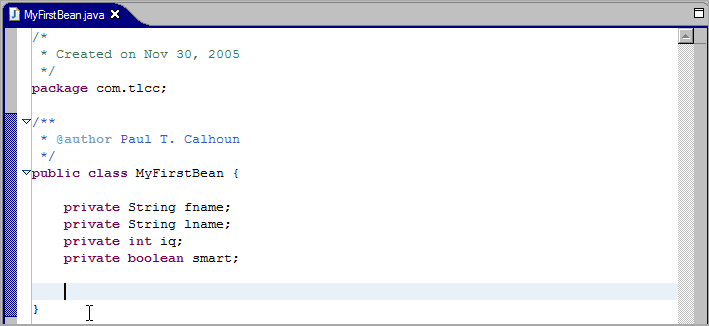
- To generate the getter and setter methods, right click in the body of the Java editor and choose Source | Generate Getters and Setters... from the context menu.
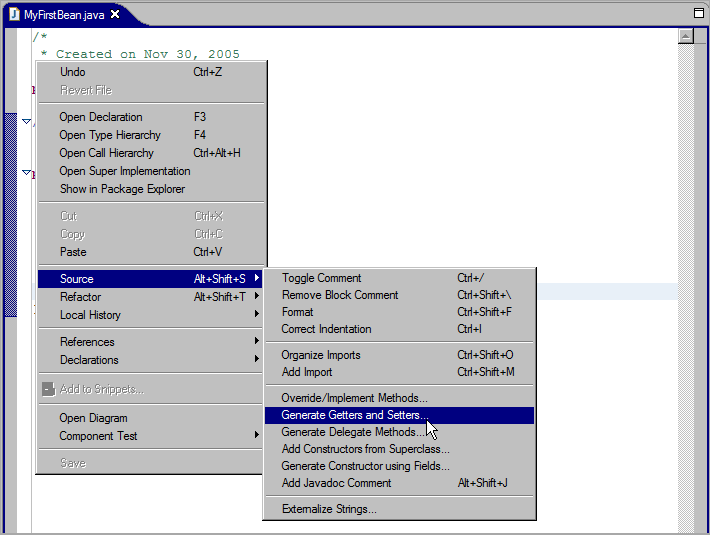
- In the Generate Getters and Setters dialog, select the methods to generate. You can click the buttons on the side to select all, deselect all, or select only the getters or setters. You may also determine the insertion point and the sort by option in this dialog box. When finished click the [OK] button to continue.
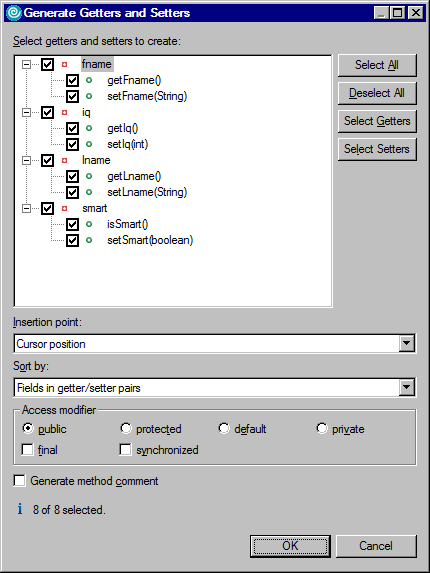
- The wizard will generate the getter and setter methods as shown in this image. Add code to complete the methods as required.
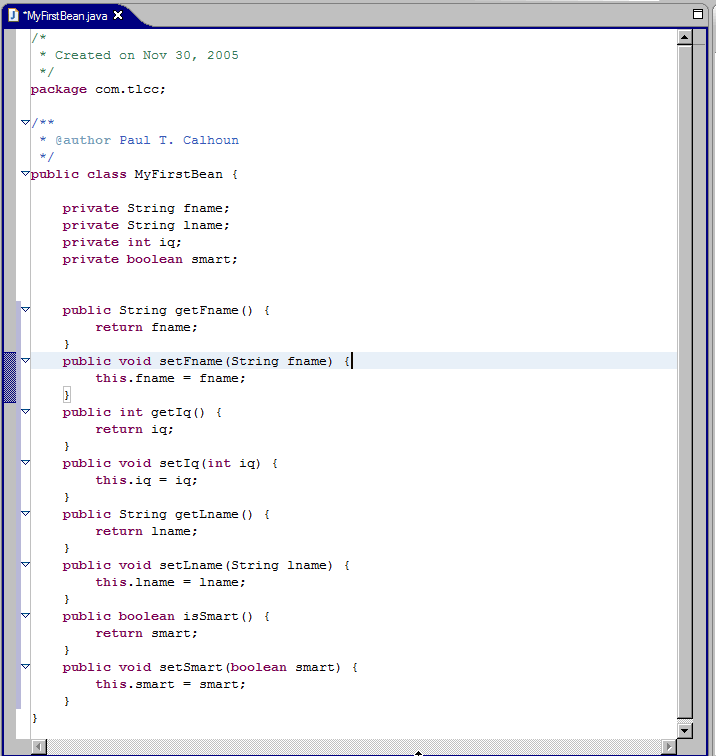
- Save the JavaBean class file.
This bean can now be used in other Java programs.
Technique: Implementing and Using a JavaBean in a Java Program
A JavaBean is used like any other Java classes in your application. The main steps to use a JavaBean are:
- Import the JavaBean class.
- Create a new instance of the class.
- Call the setter methods to update the field values.
- Call the getter methods to return the field values.
This code walkthrough shows how MyJavaClass implements and uses a JavaBean. Line 4 creates a reference to the JavaBean. Lines 5-12 use the beans setter methods to set the values of the bean. Lines 13-16 concatenate the First and Last names into a StringBuffer. Lines 17 to 19 produce the class output.
1. | package com.tlcc; |
2. | public class MyJavaClass { |
3. | public static void main(String[] args) { |
4. | MyFirstBean mb = new MyFirstBean(); |
5. | mb.setFname("Paul"); |
6. | mb.setLname("Calhoun"); |
7. | mb.setIq(100); |
8. | if (mb.getIq() > 100) { |
9. | mb.setSmart(true); |
10. | } else { |
11. | mb.setSmart(false); |
12. | } |
13. | StringBuffer fullname = new StringBuffer(); |
14. | fullname.append(mb.getFname()); |
15. | fullname.append(" "); |
16. | fullname.append(mb.getLname()); |
17. | System.out.println(fullname); |
18. | System.out.println("Their IQ is: " + mb.getIq()); |
19. | System.out.println("They are smart: " + mb.isSmart()); |
20. | } |
21. | } |
The output from running this Java class in Rational Developer is;
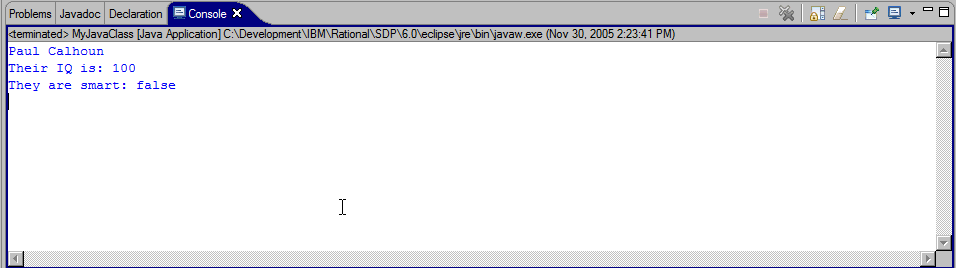
You have seen how easy it is to create and use beans in Rational Developer so use them in your Java Code whenever possible.
This tip was contributed by Paul Calhoun. Paul is TLCC's instructor for the Java, WebSphere and Rational courses. He is a noted speaker and instructor at many industry conferences. In addition to his work as a TLCC instructor and course developer Paul also is a consultant. He can be reached at pcalhoun@nnsu.com. |