Date tip published: | 09/05/2006 |
Description: | There are many actions which can be coded much easier in Java than LotusScript. Accessing web services or accessing the content on an HTML page are good examples. In addition, there are many public domain Java classes that handle common tasks. Many payment processing vendors have a Java API to process credit cards. So, what's a LotusScript coder to do? Use LS2J to call Java classes from within a LotusScript program! This tip will introduce the use of LS2J. |
To learn more about LotusScript use the following links:
Beginner LotusScript for Notes Domino 7
Intermediate LotusScript for Notes and Domino 7
Advanced LotusScript for Notes Domino 7
Using LS2J to Access Java Classes from LotusScript
Java has been the programming language of choice for the last few years. Many Java APIs (classes) have been released. For example, several of the credit card processors (like VeriSign) provide a Java API to process credit card transactions. There are also classes to enable the consumption of web services (as a client.)
Release 6 added the ability to call Java classes from LotusScript using the LS2J LotusScript Extension. This extended the world of Java to LotusScript.
Technique - Using LS2J to Access Java Classes from LotusScript
There are four main steps to access a Java class from LotusScript. Click the section headings below for details on each step.
Step 1: Provide Access to the Java Class from Notes/Domino
Java classes can be accessed from Notes/Domino in two ways:
- Access a Java Library in the Domino database
- Access Java class files from the file system
Access a Java Library in the Domino Database
Create the Java Library in the Domino database by clicking the [New Java Library] button in the Shared Code\Script Libraries list. Add or import the Java class in the Java library. An advantage of this approach is that, as a Domino design element, the Java class replicates with other copies of the Domino database. A disadvantage is that the Java library cannot be shared across databases and must exist in the Domino database from where it is accessed.
Access Java Class Files from the File System
In many cases the Java program will come distributed as a compiled program (.class or .jar file). In this case, the class file can be placed on the local hard drive of the Notes client or Domino server. When this is done, the NOTES.INI file for the client or server must be modified to add the location of the class files.
The NOTES.INI variable is "JavaUserClasses" and the syntax is:
JavaUserClasses = <classpath1>;<classpath2>; ... <classpathn>
For example:
JavaUserClasses=c:\notes7\myjava |
A disadvantage of this approach is that, since they are NOT a Domino design element, the Java class will not replicate with other copies of the Domino database. However, there can be an advantage to this approach when the Java classes only need to be accessed on a single Domino server, and not from Notes clients. In this case the Java classes can be accessed from multiple databases on the same Domino server.
Using JAR files
The ".jar" file extension must be specified when the Java classes are in a JAR file. For example:
JavaUserClasses=c:\notes7\StockQuote.jar |
|
|
Another option is to place the class or jar file in the \jvm\lib\ext directory which is located in the Notes program directory. The does not require modification of the NOTES.INI file.
Step 2: Load the LS2J LotusScript Extension
To load the LS2J LotusScript Extension, add this UseLSX statement to the Options event for the LotusScript module:
For example:
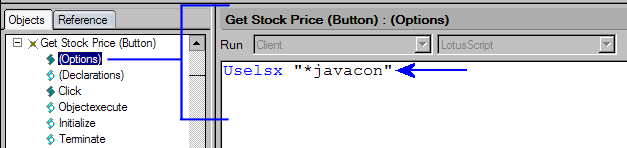
LS2J System Requirements
LS2J is implemented on all Domino platforms. Your system must meet the following requirements:
- The system must have enough memory to support both the Java Virtual Machine (JVM) and the LotusScript client applications.
- To use LS2J from within Notes, remember that your LotusScript code must include:
Uselsx "*javacon"
Note LS2J is implemented entirely as an LSX (in C++). There is no Java component to distribute. |
UseLSX statement
Loads a LotusScript extensions (lsx) file containing Public definitions needed by the module being compiled.
Syntax
UseLSX lsxLibraryName
Elements
lsxLibraryName
A string literal specifying the lsx file to load, either a name prepended with an asterisk or the full path name of the file. If you specify a name prepended with an asterisk (for example, "*LSXODBC"), the file is determined by searching the registry, initialization file, or preferences file, depending on the client platform. The Windows 95 registry, for example, might contain an entry for HKEY_LOCAL_MACHINE, SOFTWARE, Lotus, Components, LotusScriptExtensions, 2.0, LSXODBC, whose value is "c:\notes95\nlsxodbc.dll."
lsxLibraryName can contain "?", an optional flag that signals the lsx file is not necessary during run time. The question mark is part of the String, * and % flags follow the ? if desired. e.g. "?*NAME_OF_LIBRARY". For example:
const lsxLibraryName = "?NAME_OF_LIBRARY"
UseLSX lsxLibraryName
Usage
LotusScript registers the Public classes defined in the lsx file for use in the module containing the UseLSX statement. Other modules that use this containing module can also access these Public classes.
Note that Lotus Notes supports the UseLSX statement. The UseLSX statement loads a .LSX file containing Public definitions. These definitions then become available to the current script. Once the .LSX file has been downloaded, its classes are browsable in the Notes class browser.
The Notes platform has a registry of LSXes. If the file-specification string in the UseLSX statement begins with an asterisk (*), then Notes looks in the registry for the name consisting of the rest of the string. The registry entry for that name specifies the file location in the platform file system.
The "_" is reserved for Notes specific dlls. This is a change put in as of Notes 4.5.1. If you attempt to load a dll in Notes 4.51 or greater using LotusScript and the name of the dll is preceded by an underscore you will receive the error "Error in loading DLL".
A library name prefixed with a '?' is considered to be optional at run time. The library must be present at compile time in order to compile the script, however, if the LSX cannot be loaded at run time, the script will still execute as long as classes defined by the LSX are not referenced or functions/procedures defined by the LSX are not called from the script. If the LSX is not loaded and a line of script references an LSX class or procedure, the following errors would be thrown.
ERR = 230
ERROR = Unknown class instance
ERR = 48
ERROR = Error in loading DLL |
Step 3: Connect to the Java Library
This step is only required when accessing Java classes in a Java Library. Skip this step when the Java class files are accessed from the file system.
To connect to the Java library, add this Use statement to the Options event for the LotusScript module:
For example, this image shows how to connect to the "StockQuote799" Java Library:
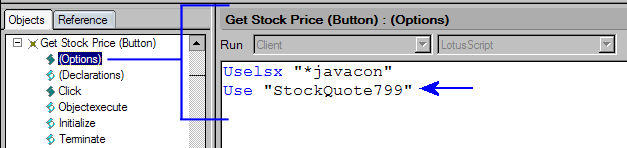
From Lotus Domino Designer 7 Help |
|
Use statement
Loads a module containing Public definitions needed by the module being compiled.
Syntax
Use useScript
Elements
useScript - A String literal, or a constant containing a String value, specifying the module to load.
The Lotus software application that you’re using determines whether useScript must be compiled before use. Consult the product documentation for more information.
Usage
The Use statement can appear only at module level, before all implicit declarations within the module. Note that the Use statement is supported in Lotus Notes. |
|
Step 4: Call the Java Class Methods from LotusScript Code
The key classes in the LS2J LotusScript Extension are JavaSession, JavaClass and JavaObject. The following image highlights the use of these classes.
Use the JavaSession class to establish a connection from LotusScript to the Java Virtual Machine (JVM). For example:
Dim mySession As JAVASESSION |
Set mySession = New JavaSession() |
Once a JavaSession object is instantiated, create a JavaClass object for the Java class to use. Use the getClass method of the JavaSession class to create the JavaClass object. Indicate the name of the external Java class file or the name of the Java library when creating the JavaClass object. In this example, the name of the Java library is "StockQuote799":
Dim jclass As JAVACLASS |
Set jclass = mySession.getClass("StockQuote799") |
Create a JavaObject instance for the class and define the data type and parameters to pass to the method in the class. Use the createObject method of the JavaClass class to create the JavaObject instance. Since LotusScript and Java have different data types, you must define the data types for the Java class in the createObject method. The types of data to pass are indicated in the first argument of createObject. The first argument is known as the "signature" argument. In the remaining arguments (up to 12), pass the values for the arguments for the class. This table provides examples of Java class constructors and their corresponding signature arguments:
Java class constructor | Signature argument for CreateObject | Description |
myjava(int i) | "(I)V" | One input parameter, primitive data type |
myjava(float i,float j ) | "(FF)V" | Two input parameters, both primitive data type |
myjava(String s1,String s2 ) | "(Ljava/lang/String;Ljava/lang/String;)V" | Two input parameters, both String data types (Precede the Java definition with a "L" and end each one with a semi-colon.) |
myjava(String s1,int i,String s2 ) | "(Ljava/lang/String;I;Ljava/lang/String;)V" | Three input parameters, a String data type, an integer (note the "I") and a String data type |
The "V" on the end means the object will return nothing (void).
In this example, a single string-type argument is passed as indicated by "(Ljava/lang/String;)V" in the first createObject argument.
Dim jobj As JavaObject |
Set jobj = jclass.createObject("(Ljava/lang/String;)V", doc.stock(0)) |
Finally, call the method in the Java class by name. In this example the getQuote method is called from the StockQuote799 Java library and the returned vale is assigned to the Price field in the document:
doc.price = jobj.getQuote() |
LS2J classes
With LS2J, Lotus introduces the concept of a Java object reference. Similar to an OLE object reference, it is not a predefined class; rather, it represents a runtime instance of a Java object. Its properties and methods are determined at run time.
The following table lists the LS2J interface classes.
LS2J class | Description |
JavaClass | Represents a Java class. |
JavaError | Allows LotusScript programmers to find an error raised from the Java program. |
JavaMethod | Contains information about Java methods in a class. |
JavaMethodCollection | Is the enumerator for all the methods in a Java class. |
JavaObject | Represents a Java object instance. This is the key to connecting with a Java object. |
JavaProperty | Contains information about a Java property in a class. |
JavaPropertyCollection | Is the enumerator for all the properties in a Java class. |
JavaSession | Represents a connection instance of JVM with which LotusScript is interfacing. |
|
getClass method
This method returns a reference to a Java class.
Defined in
JavaSession
Syntax
Set JavaClass = javasession.getClass(ClassName$)
Parameters
ClassName$ - String. The name of the class you would like to retrieve. For example, "java/lang/Integer."
Usage
This method will return a Java class reference with which a Java object can be created within LotusScript.
|
createObject method
This method creates a JavaObject instance base of the JavaClass object.
Defined in
JavaClass
Syntax
Set javaobject = javaclass.CreateObject(Signature[, Argument1, ..., Argumentn])
Parameter
Signature - String. This is a JNI signature representing the constructor to use to initialize the object.
JNI Signature | Description | Examples | Constructor requires |
B | byte | (B)V | A byte argument |
C | char | (C)V | A char argument |
D | double | (D)V | A double argument |
F | float | (F)V | A float argument |
I | int | (I)V | An int argument |
J | long | (J)V | A long argument |
S | short | (S)V | A short argument |
Z | boolean | (Z)V | A Boolean argument |
L<fully-qualified-class> | fully -qualified class | "(Ljava/lang/Integer;)V"
A java.lang.Integer argument |
[<sigtype> | Array of <sigtype> | ([I)V | An int array |
L<fully-qualified-class>;
L<fully-qualified-class>;
I
L<fully-qualified-class>; | fully-qualified class
fully-qualified class
int
fully-qualified class | "(Ljava/lang/String;Ljava/lang/String;ILjava/lang/String;)V"
4 arguments:
java.lang.String,
java.lang.String,
int,
java.lang.String |
If the constructor has no parameters, call CreateObject with no parameters:
<javaclass>.CreateObject()
If the constructor has one or more parameters, call CreateObject with a signature parameter as follows:
<javaclass>.CreateObject("(...)V")
where ... represents the types of one the parameters in the table. Note that each signature for a fully-qualified-class must start with an L and end with a semicolon.
Argumentn - The arguments needed by the constructor, varying from 0 to 12. These arguments are optional.
Usage
This method creates a JavaObject instance base of the JavaClass object, and returns a JavaObject reference. By default, the empty constructor is used. Otherwise, the user must specify which constructor by using the signature.
Error thrown
LS2J error if there are any issues regarding the signature or the arguments.
|
Using LS2J to Access Java Classes from LotusScript
This demonstration shows how a button which is coded with LotusScript can invoke a method in a Java library. This demonstration has a Java class which gets the stock price for a stock.
Note that this Java class has hardcoded stock values for three stocks, IBM, MSFT, and JNJ. This class simulates Java code that could be written to use web services to actually obtain a stock quote. For more information on writing Java web services go to the following article on DeveloperWorks at IBM's website:
http://www-128.ibm.com/developerworks/lotus/library/domino-webservices/
- Detach the database below to your Notes data directory by clicking on the database icon:

- Open the LS2J Demonstration database.
- Create a new document with the Demo 1.8 Calling Java from LotusScript form. Follow the demonstration steps continued on the form.
- Continue to the Technique section below.
|
|
Technique
Here is a description of the code for the [Get Stock Price] button in the demonstration.
These three lines of code are from the (Options) event for the button. The LS2J LotusScript Extensions are loaded in Line 2. In Line 3, the StockQuote799 Java library is accessed.
1. | Option Declare |
2. | Uselsx "*javacon" |
3. | Use "StockQuote799" |
The rest of the code is from the Click event for the button.
The first set of statements declare and set variables for the current workspace, the front-end document and the back-end document.
1. | Sub Click(Source As Button) |
2. | Dim ws As New NotesUIWorkspace |
3. | Dim uidoc As NotesUIDocument |
4. | Set uidoc = ws.CurrentDocument |
5. | Dim doc As NotesDocument |
6. | Set doc = uidoc.Document |
These two statements declare and instantiate a JavaSession object, mySession.
7. | Dim mySession As JAVASESSION |
8. | Set mySession = New JavaSession() |
A JavaClass object, jclass, is declared and instantiated. The jclass object is set to access fields and methods in the "StockQuote799" Java library.
9. | Dim jclass As JAVACLASS |
10. | Set jclass = mySession.getClass("StockQuote799") |
A JavaObject object, jobj, is declared in Line 11 and instantiated in Line 12 using the createObject method of the JavaClass class. In this example, a single string-type argument (i.e. doc.Stock(0)) is passed as the second argument in the CreateObject method. The first argument passed to CreateObject indicates the type of data for the argument which is passed. In this example, a single string-type argument is passed so the first argument of CreateObject is "(Ljava/lang/String;)V". Line 13 calls the getQuote() Java method to retrieve the stock price.
11. | Dim jobj As JavaObject |
12. | Set jobj = jclass.CreateObject("(Ljava/lang/String;)V", doc.stock(0)) |
13. | doc.price = jobj.getQuote() |
14. | End Sub |
|
|
Terminology - Property same as Field
The Lotus Domino Designer 7 Help uses the term property and many of the methods and properties of the LS2J classes use property as part of their name. Examples would be the JavaProperty class and the getProperty method in the JavaClass class.
Some Java programmers may be more familiar with the term "fields." These are the same as properties. A field is a variable declared in a class. If this variable is public then the field's value can be accessed and set. Field and property can be thought as being the same for the discussions in this lesson and in Lotus Domino Designer 7 Help. |
|
Accessing Java Properties and Methods using getProperty and getMethod
In the example above, a Java method was called by referencing its name using dot notation syntax. The dot notation syntax can also be used to set a Java property.
Dot Notation Syntax to Access Java Properties and Methods
Use this dot notation syntax to call a Java method:
javaobject.javamethodname() which will return a value to LotusScript.
Use this dot notation syntax to set a Java property:
javaobject.javamethodname = (some LotusScript value) |
|
However, there are several situations when the dot notation syntax may not work. Instead, the getProperty and getMethod methods of the JavaClass class must be used to access the property or method. Failure to use this approach may result in ambiguous results or a run-time error.
There are three situations when the dot notation syntax may not work:
- Java is case sensitive and LotusScript is not. Java allows the use of two methods that differ only by the case of the name. Since LotusScript is not case sensitive, it has no way to differentiate the two different Java methods using the dot notation syntax.
- A Java method or property name is greater than 40 characters. LotusScript does not allow for more than 40 characters for a method name, Java does.
- Java allows for more than one method with the same name. LotusScript does not. The way Java differentiates between these methods is by comparing the input parameters (signature). There could be two Java methods called getStockQuote which differ only by the input parameter's data type. One getStockQuote requires a String input parameter and the other an integer value:
getStockQuote(String s)
getStockQuote(integer i)
The method that gets executed will depend on the data type passed. getStockQuote("IBM") would run the first method and getStockQuote(345) would run the second. This is called overloading in Java and there is no equivalent in the LotusScript language.
If one of the above three situations apply, do not use the dot notation syntax. Instead, use getProperty or getMethod method to access the property or method in the Java class.
Technique - Using the getProperty Method
Use the getProperty method of the JavaClass class to return a specified property in the form of a JavaProperty object.. The getProperty method takes the name of the property to return as an argument. This argument is case sensitive and can be greater that 40 characters.
From Lotus Domino Designer 7 Help |
|
getProperty method
This method returns a property.
Defined in
JavaClass
Syntax
Set Javaproperty = javaclass.getProperty(PropertyName)
Parameter
Propertyname - String. Case sensitive. Name of the property you want a handle of.
Usage
This method returns the property matching Propertyname. |
|
The getProperty method returns the specified property in the form of a JavaProperty object. To get and set the property values, use the getValue and setValue methods of the JavaProperty class.
getValue method
This method returns the JavaProperty value.
Defined in
JavaProperty
Syntax
Set Variant = javaProperty.getValue([JavaObject])
Parameter
JavaObject - JavaObject. The instance of an object from which you want a property value, if the property is not static. Optional if the property is static.
Return value
Variant - Value of the JavaProperty.
Usage
This method is used to get the value of either a public static property or a public object property. The object is necessary if the property is not static, and disregarded if the property is static. |
setValue method
This method sets the JavaProperty value.
Defined in
JavaProperty
Syntax
Call javaproperty.setValue(NewValue [, JavaObject])
Parameters
NewValue - Variant. New Value for the JavaProperty.
JavaObject - JavaObject. Object to be set, if the property is not static. Optional if the property is static.
Error thrown
IllegalAccessException. Thrown if the value is of the wrong type, or if the property is read only. |
Technique - Using the getMethod Method
Use the getMethod method of the JavaClass class to return a specified method in the form of a JavaMethod object. The getMethod method takes two arguments. The first argument is the name of the property to return, is case sensitive and can be greater that 40 characters. The second argument indicates the signature of the method to return.
From Lotus Domino Designer 7 Help |
|
getMethod method
This method returns a method from a JavaClass object.
Defined in
JavaClass
Syntax
Set Javamethod = javaclass.GetMethod(Methodname, Signature)
Parameters
Methodname - String. Case sensitive. Name of the method you want a handle of.
Signature - String. JNI Signature representing the method needed.
JNI Signature | Description |
B | byte |
C | char |
D | double |
F | float |
I | int |
J | long |
S | short |
V | void |
Z | Boolean |
L<fully-qualified-class> | fully-qualified class |
[<sigtype> | Array of <sigtype> |
Usage
This method returns the method matching the name given with the specified signature.
Error thrown
NoSuchMethodException if the Java method does not exist with the signature given. |
|
The getMethod method returns the specified method in the form of a JavaMethod object. To invoke the method, use the Invoke method of the JavaMethod class.
From Lotus Domino Designer 7 Help |
|
Invoke method
This method executes a method.
Defined in
JavaMethod
Syntax
Set Variant = javamethod.Invoke([JavaObject [,Argument1...[, Argument12]])
Parameters
JavaObject - JavaObject. The instance of an object, if the method is not static. Optional if the method is static.
Argumentn - Variant. Optional. The arguments needed by the method. Maximum of 12 arguments.
Return value
Variant. Result of the invoked method. |
|
|